How to Create a Grid of Divs Using JavaScript
JavaScript serves as a powerful tool for dynamically generating a grid containing div elements. This article will introduce the basic concepts of creating such a grid, explore its practical applications, and provide usage guidelines.
In web development, it's often necessary to create grid layouts to display and organize information. JavaScript serves as a powerful tool for dynamically generating a grid containing div elements. This article will introduce the basic concepts of creating such a grid, explore its practical applications, and provide usage guidelines.
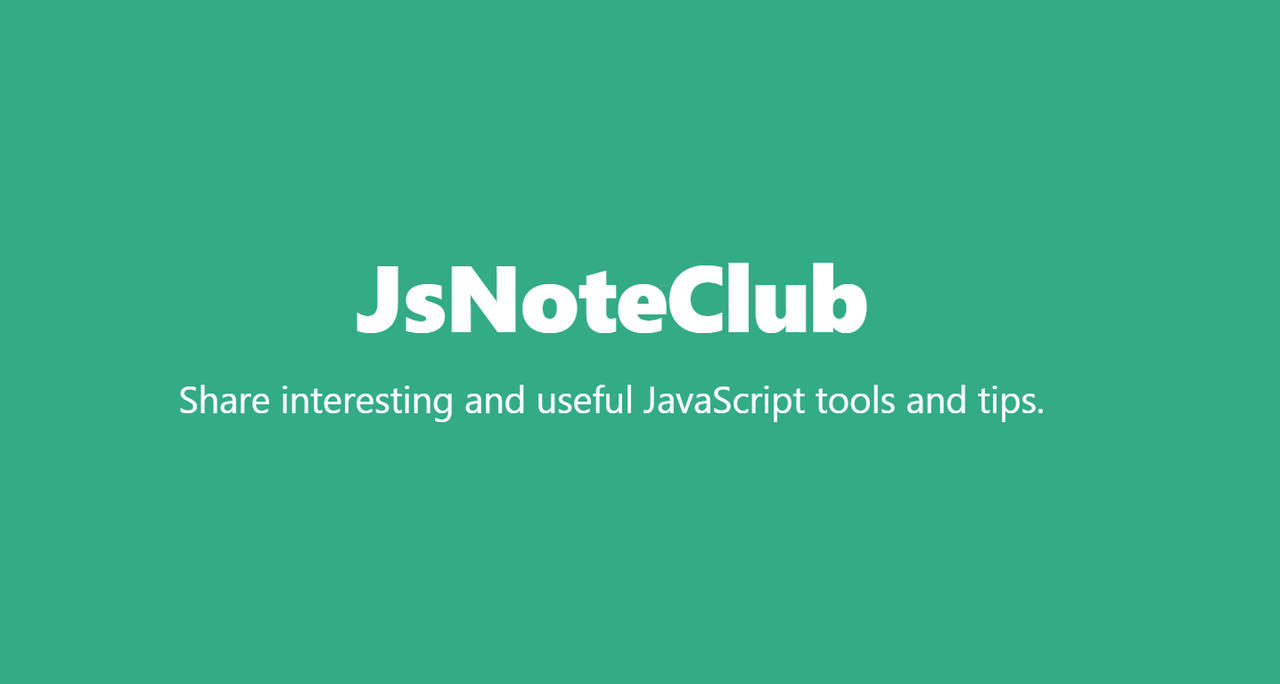
Application Scenarios for Div Grids
- Image Library Display: In a webpage showcasing an image library, use a grid to display thumbnails, allowing users to quickly browse and select images.
- Product Catalog: On an e-commerce website, present products in a grid layout for easy navigation through multiple items.
- Calendar Application: Display dates and events in a grid format within a calendar application to provide clear organization of time.
- Dashboard Layout: In a management dashboard, organize different information panels using a grid layout to enhance user experience.
Best Practices
Below are the steps to create a simple grid.
Step 1: Create HTML File
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Grid Example</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<script src="script.js"></script>
</body>
</html>
Step 2: Create CSS File (styles.css)
body {
margin: 0;
padding: 0;
display: flex;
flex-wrap: wrap;
}
.grid-item {
box-sizing: border-box;
width: 100px;
height: 100px;
border: 1px solid #000;
}
Step 3: Create JavaScript File (script.js)
document.addEventListener("DOMContentLoaded", function() {
// Get the body element
var body = document.body;
// Create a grid, for example, 5x5
var rows = 5;
var columns = 5;
// Loop to create grid items and append them to the body
for (var i = 0; i < rows; i++) {
for (var j = 0; j < columns; j++) {
var gridItem = document.createElement("div");
gridItem.classList.add("grid-item");
// Set the position of each grid item
gridItem.style.left = j * 100 + "px";
gridItem.style.top = i * 100 + "px";
// Append the grid item to the body
body.appendChild(gridItem);
}
}
});
Step 4: Run the Code
Save the above code into respective files (HTML, CSS, JavaScript) and open the HTML file in a web browser. You will see a page with a 5x5 grid, where each grid item is sized at 100x100 pixels.You can adjust the number of rows, columns, and the size of each grid item as needed. This example serves as a basic starting point, and you can customize it further based on the requirements of your project.
Tips, Tricks, and Considerations
- Dynamic Grid Modification: You can dynamically modify created grids by listening to user interactions or other events using JavaScript.
- Grid Style Customization: Depending on design requirements, you can customize the style of the grid, such as colors and spacing.
- Performance Optimization: When creating large grids, consider performance optimization to ensure a smooth user experience.
Conclusion
Creating a div grid using JavaScript is a common and useful task applicable to various scenarios. Whether showcasing a gallery, designing layouts, or visualizing data, dynamic grid layouts offer flexible solutions. By skillfully leveraging CSS Grid, diverse grid layouts can be easily achieved, enhancing the convenience of web development.
Reference Links:
Learn more:
- 30 JavaScript One-Liners to Turn You into a JavaScript Wizard
- 5 Methods of Creating Objects in JavaScript
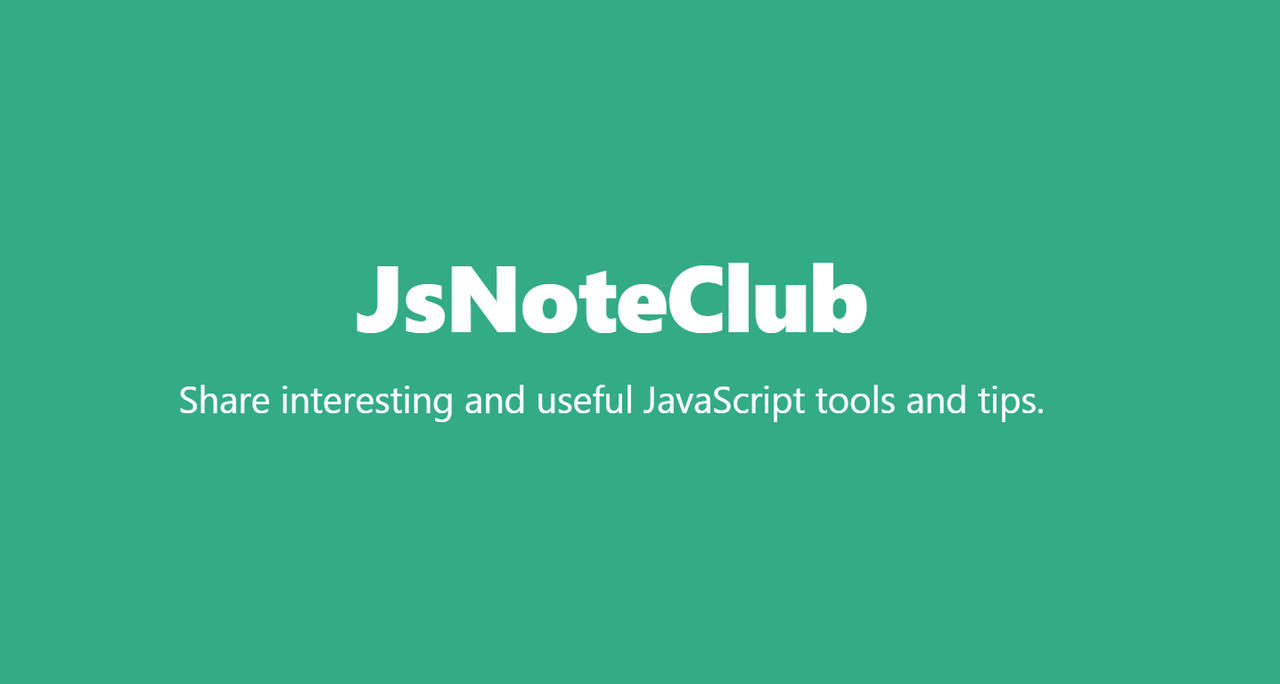
JsNoteClub:Share interesting and useful JavaScript tools and tips.