5 Methods of Creating Objects in JavaScript
JavaScript offers five methods for creating objects, including object literals, new Object() syntax, constructor functions, Object.create() method, and ES6 class syntax. Each method suits different scenarios, providing flexibility for efficient and maintainable code.
In JavaScript, objects are versatile tools that can be created in various ways, each suitable for different scenarios. Knowing when to use each method is key to writing efficient and maintainable JavaScript code. Let's explore the five common methods of creating objects in JavaScript and delve into the best use cases for each method.
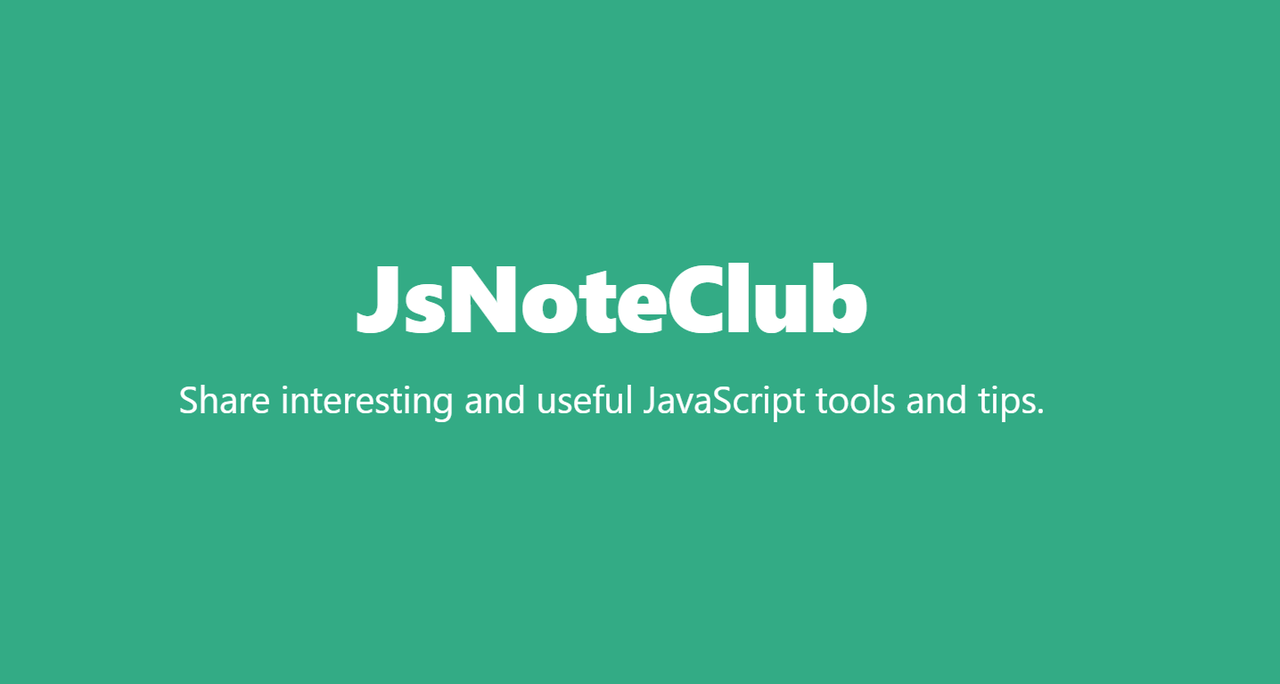
Object Literals
Object literals are the simplest and fastest way to create objects in JavaScript using curly braces {}. This method is ideal for creating individual, standalone objects that don't require blueprints or repeated instantiation.
const car = {
make: 'Toyota',
model: 'Corolla',
year: 2021
};
console.log(car);
Use object literals for quick and straightforward objects when you don't need methods or prototypes. They are excellent for configuring options, storing data, and encapsulating related properties and methods in a simple structure.
New Object() Syntax
Using the new Object()
syntax is a more explicit way of creating objects in JavaScript. Similar to object literals but more readable, especially for those familiar with object creation in other programming languages.
const person = new Object();
person.name = 'John';
person.age = 30;
person.isEmployed = true;
console.log(person);
This method is useful when you want to explicitly demonstrate object creation or when transitioning from languages that heavily use class-based or constructor-based object creation. It's handy when dynamically adding properties based on conditions.
Constructor Functions
Constructor functions are used to create multiple instances of similar objects, serving as blueprints for creating objects of the same type in JavaScript.
function Smartphone(brand, model, year) {
this.brand = brand;
this.model = model;
this.year = year;
}
const myPhone = new Smartphone('Apple', 'iPhone 13', 2021);
console.log(myPhone);
Constructor functions are ideal when you need multiple objects with similar properties and methods. They are well-suited for creating entities like users, products, or other entities sharing a common structure but with different values.
Object.create() Method
Object.create()
in JavaScript creates a new object with a specified prototype and properties. This method provides more control over object inheritance compared to other methods.
const animal = {
type: 'Animal',
displayType: function() {
console.log(this.type);
}
};
const dog = Object.create(animal);
dog.type = 'Dog';
dog.displayType(); // Output: Dog
Use this method when you need to create an object directly inheriting from another object without invoking the parent constructor function. It's a powerful tool for complex inheritance structures, behavior delegation, and other advanced patterns.
ES6 Class Syntax
ES6 classes offer a more traditional, class-based approach to creating objects in JavaScript. It is syntactic sugar for prototype-based inheritance, providing a clearer and more familiar syntax for those coming from class-based languages.
class Book {
constructor(title, author, year) {
this.title = title;
this.author = author;
this.year = year;
}
getSummary() {
return `${this.title} was written by ${this.author} in ${this.year}`;
}
}
const myBook = new Book('1984', 'George Orwell', 1949);
console.log(myBook.getSummary());
Use ES6 classes for more complex applications where code organization, readability, and inheritance structure are crucial. They are beneficial for large applications and working in teams that require clear and standardized coding practices.
Which Method is Best and Fastest
There is no one-size-fits-all answer for the best and fastest method for creating objects because it largely depends on the specific requirements and context of the application.However, for simplicity and speed, object literals are the fastest and simplest way to create objects, especially for straightforward one-time use objects. For more complex and structured applications, ES6 classes strike a balance between readability, traditional syntax, and performance, although they may have slight overhead compared to constructor functions.
Conclusion
Choosing the right method for creating objects in JavaScript depends on the context and specific requirements of the application. Whether it's a simple one-time use object or a complex structure requiring inheritance, understanding these five methods can help you write more efficient and maintainable JavaScript code.
Learn more:
- How to Create Empty Functions in JavaScript
- How to Call JavaScript Functions in HTML Without Using onclick
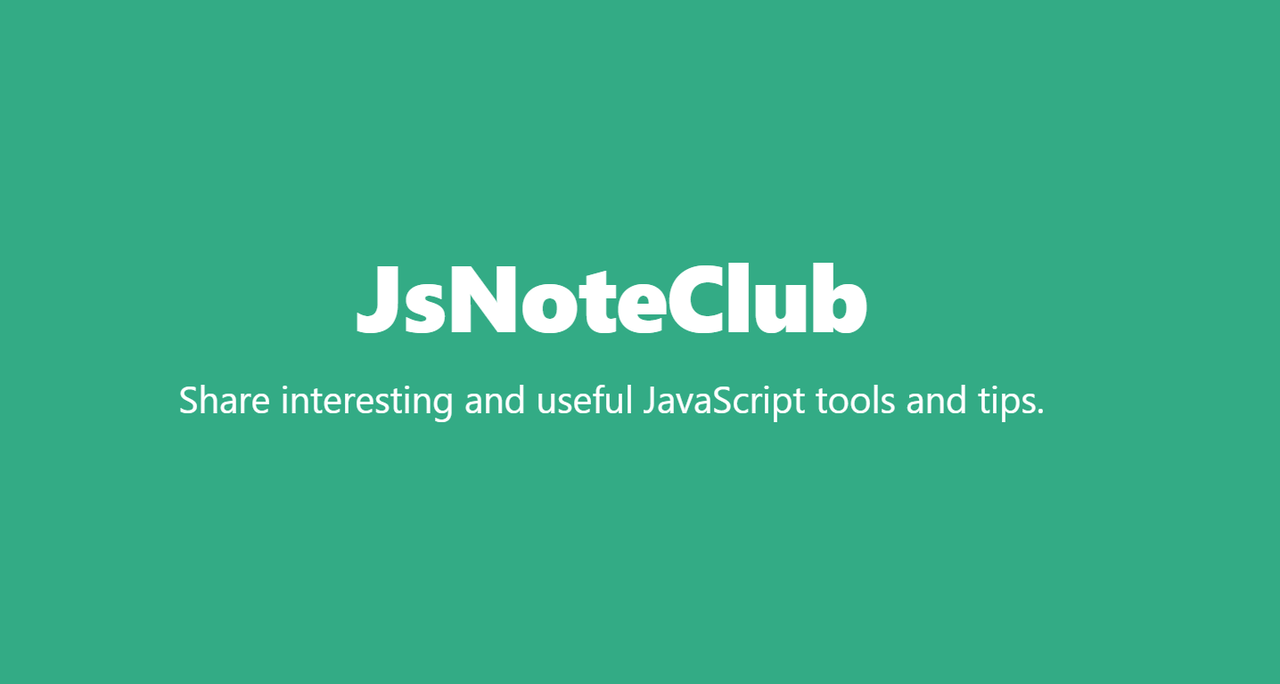
JsNoteClub:Share interesting and useful JavaScript tools and tips.