How to Create Empty Functions in JavaScript
This article explores the creation and practical applications of empty functions in JavaScript. Learn various methods, use cases, and engage in interactive exercises to enhance your understanding of this versatile coding concept.
In JavaScript, creating empty functions is a common requirement, especially when defining placeholder functions or leaving interfaces for future implementations. An empty function is a type of function that doesn't execute any operations, but it plays a crucial role in code structure and design. This article explores how to create empty functions in JavaScript, along with their practical applications, functionality overview, syntax, and usage. Practical examples with detailed explanations and interactive exercises are provided to enhance understanding.
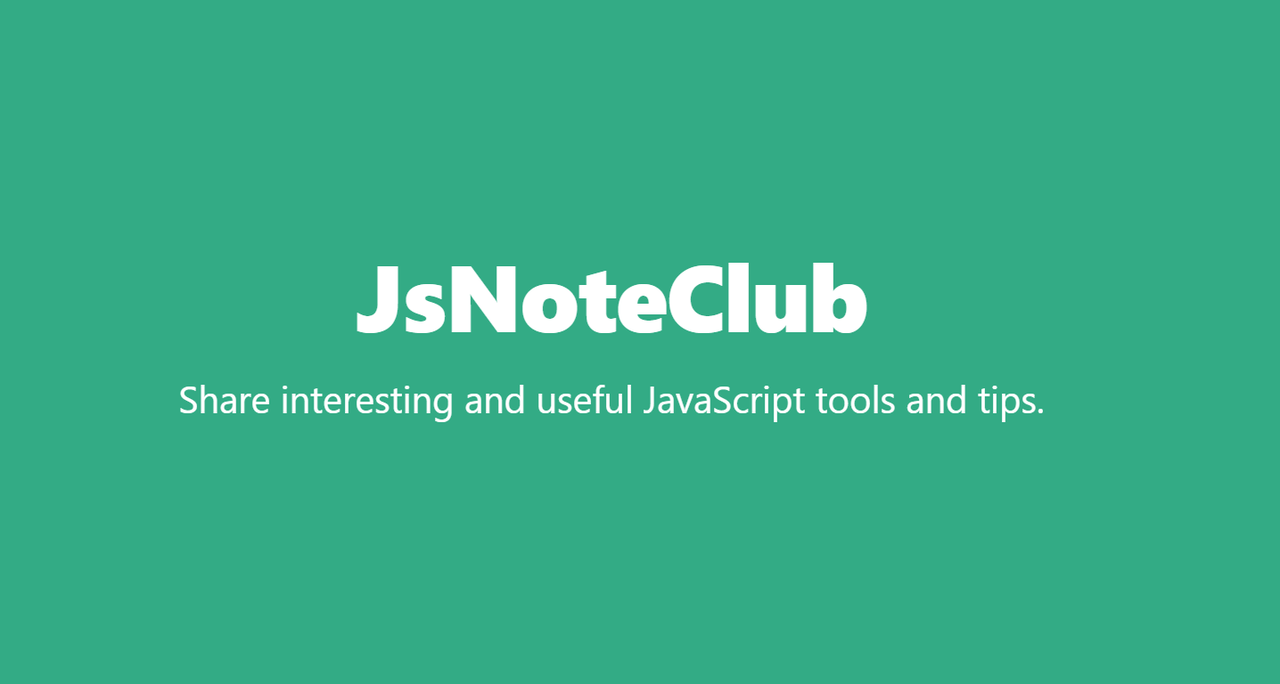
Empty Functions Use Cases
1. Placeholder Functions
During project development, there might be a need to define interface functions that are not implemented in the initial stages. In such cases, empty functions can serve as placeholders, maintaining the integrity of the code structure.
2. Event Handler Initialization
When registering handler functions for events, but the event doesn't require handling in certain situations, empty functions can be used as default event handlers.
3. Optional Callback Functions
In some functions, optional callback parameters may exist. Using empty functions as defaults can handle cases where callbacks are not provided, eliminating the need for constant checks.
Empty Functions Syntax and Usage
In JavaScript, there are several methods to create empty functions:
1. Using an empty function body
function emptyFunction() {
// Do nothing
}
2. Using arrow functions
const emptyFunction = () => {};
3. Using the Function constructor
const emptyFunction = new Function();
Empty Functions Practical Examples
Sample Code
Let's delve into how to create empty functions in JavaScript through a practical example. Assume we have a simple data processing module where a function takes a data array and a processing function, applying the function to each element of the array. We want to provide a default processing function to ensure the function works seamlessly even when a custom processing function is not provided.
// Data processing module
function processData(data, customHandler) {
const handler = customHandler || defaultHandler;
data.forEach(item => {
handler(item);
});
}
// Default empty processing function
function defaultHandler(item) {
// Do nothing by default
}
// Using the data processing module
const myData = [1, 2, 3, 4, 5];
// Provide a custom processing function
processData(myData, item => {
console.log(`Custom handling: ${item}`);
});
// Using the default processing function
processData(myData); // Does nothing by default
In this example, the defaultHandler
function serves as an empty function, not executing any actual operations by default. When no custom processing function is provided, the processData
function uses the default processing function, ensuring the robustness and flexibility of the code.
Interactive Exercises
Suppose we have a function called calculate
that takes two numbers and an operation function, applying the operation to these numbers. To prevent errors when no operation function is provided, we can use an empty function as the default operation function. Please complete the following exercise:
// Define the calculate function
// Provide a custom operation function
calculate(5, 3, (a, b) => {
console.log(`Custom calculation: ${a + b}`);
});
// Using the default operation function
calculate(5, 3); // Does nothing by default
Reference Solution
// Define the calculate function
function calculate(a, b, operation = defaultOperation) {
operation(a, b);
}
// Default empty operation function
function defaultOperation() {
// Do nothing by default
}
// Provide a custom operation function
calculate(5, 3, (a, b) => {
console.log(`Custom calculation: ${a + b}`);
});
// Using the default operation function
calculate(5, 3); // Does nothing by default
Through this exercise, you've learned how to use an empty function as the default operation function, ensuring that the code works correctly even when no custom operation function is provided.
Tips, Tricks, or Considerations
- Empty functions are often used to provide default implementations or act as placeholders, ensuring code stability in different scenarios.
- When using empty functions as default values, pay attention to default parameter syntax to ensure the default function works when not provided.
- Flexibly apply empty functions to make your code more extensible and maintainable.
By engaging in practical examples and interactive exercises, it is expected that you now have a more in-depth understanding of creating empty functions in JavaScript and their real-world applications.
Summary
This article covered methods for creating empty functions in JavaScript, including their use cases, functionality overview, syntax, and usage. Empty functions play a vital role in code design, serving as placeholders, providing default implementations, or handling optional callbacks. Through practical examples and interactive exercises, readers should now have a deeper understanding of the concept and practical application of empty functions.
Reference Links:
Learn more:
- How to Call JavaScript Functions in HTML Without Using onclick
- How to Iterate Table Rows in JavaScript
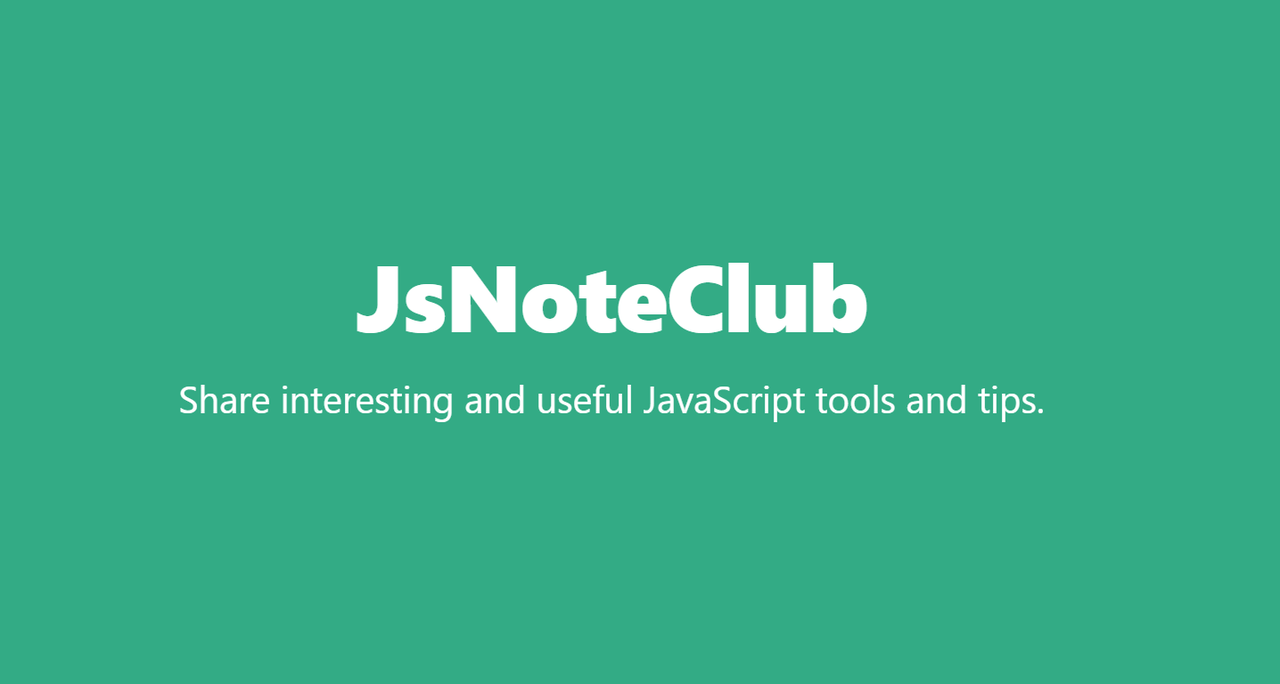
JsNoteClub:Share interesting and useful JavaScript tools and tips.