How to Iterate Table Rows in JavaScript
HTML tables are defined with the <table> tag, containing rows <tr> and cells <td> tags. To iterate over rows in JavaScript, you can use document.querySelectorAll and forEach loops.
HTML tables are defined with the <table>
tag, containing rows <tr>
and cells <td>
tags. To iterate over rows in JavaScript, you can use document.querySelectorAll
and forEach
loops.
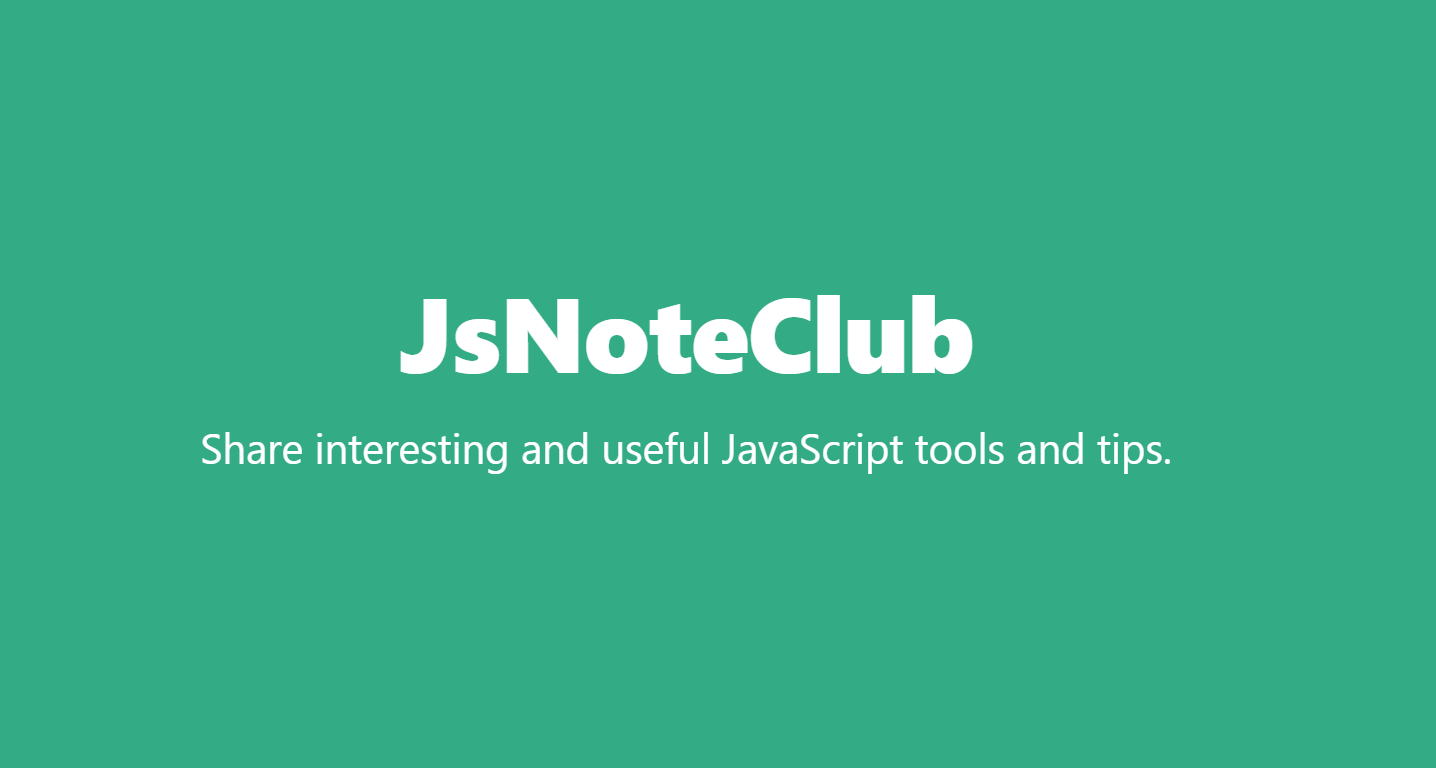
Iterate Table Rows Use Cases
Common use cases for iterating over table rows:
- Collecting table data, converting to JavaScript objects or arrays
- Applying style changes to rows like adding background colors
- Showing/hiding rows based on cell contents
Function Overview
To iterate table rows, first get the table element, use querySelectorAll
to get the <tr>
row elements, then you can loop through the rows with forEach
.
Syntax and Usage
Get table element:
var table = document.getElementById("myTable");
// or
var table = document.querySelector("table");
Get row elements:
var rows = table.querySelectorAll("tr");
Iterate rows:
rows.forEach(function(row) {
// do something with row
});
Can also use a for loop:
for (var i = 0; i < rows.length; i++) {
var row = rows[i];
// do something with row
}
Iterate Table Rows Examples
<table id="scoreTable">
<tr><td>John</td><td>80</td></tr>
<tr><td>Jane</td><td>90</td></tr>
</table>
<script>
var table = document.getElementById("scoreTable");
var rows = table.querySelectorAll("tr");
rows.forEach(function(row) {
row.style.backgroundColor = "grey";
});
</script>
The above iterates all rows and sets background color to grey.
Interactive Exercise
Output content of each row in table below:
<table id="dataTable">
<tr><td>Name 1</td><td>Data 1</td></tr>
<tr><td>Name 2</td><td>Data 2</td></tr>
</table>
<script>
// your code here
</script>
Reference Answer:
var table = document.getElementById("dataTable");
var rows = table.querySelectorAll("tr");
rows.forEach(function(row) {
var cols = row.querySelectorAll("td");
console.log(cols[0].textContent, cols[1].textContent);
});
Tips
- Get cell data with
querySelectorAll
on<td>
elements - Use
textContent
for text contents - Can use
for
loop instead offorEach
Conclusion
After getting row elements, you can use querySelectorAll
and forEach
to iterate over each <tr>
and access table data in JavaScript.
References
- https://developer.mozilla.org/en-US/docs/Web/API/Element/querySelectorAll
- https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/forEach
Learn more:
- 8 Awesome JavaScript String Manipulation Techniques
- 11 Useful JavaScript Tips to Boost Your Efficiency
- How to Check if a String is a Number in JavaScript
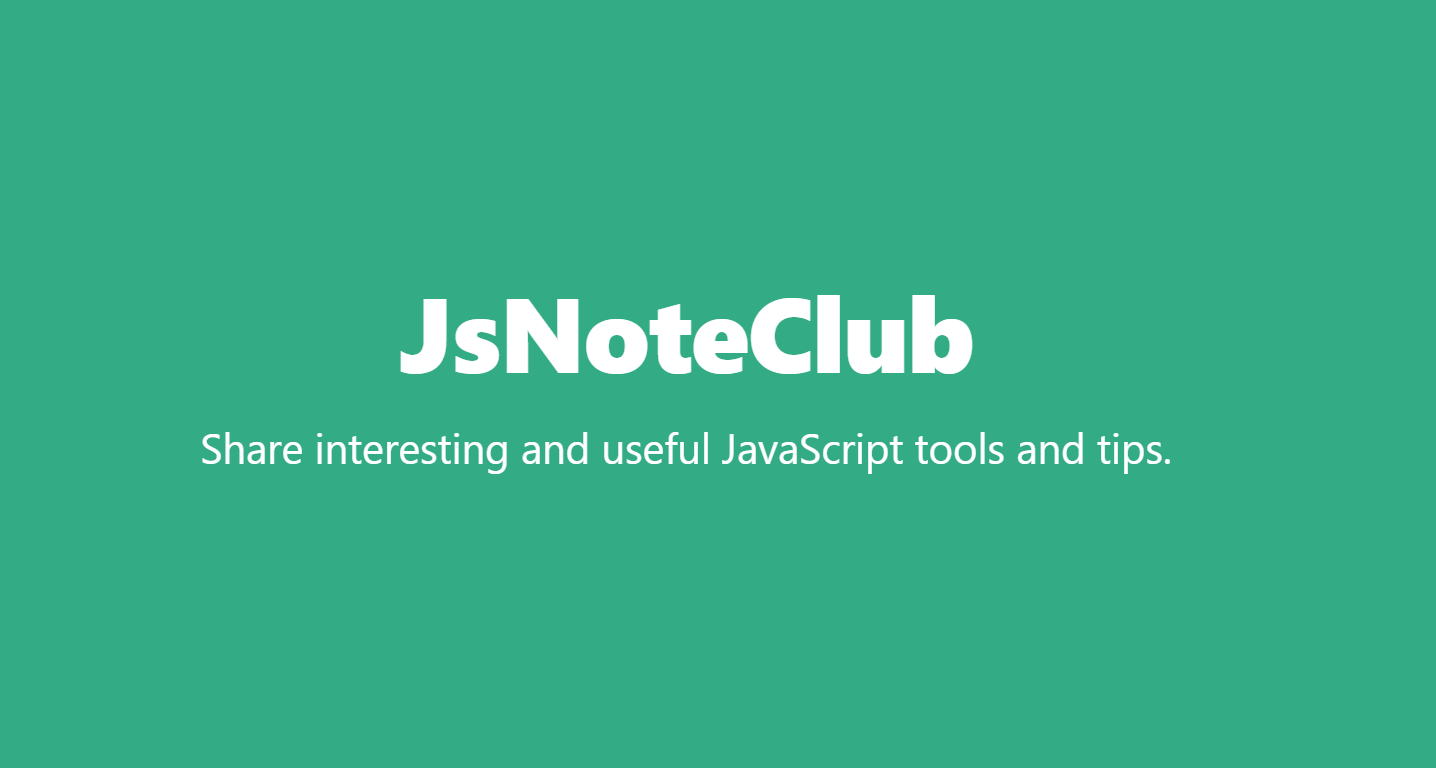
JsNoteClub:Share interesting and useful JavaScript tools and tips.