8 Awesome JavaScript String Manipulation Techniques
When working with JavaScript strings, there are many interesting techniques that can enhance our coding efficiency. In this article, I will introduce some JavaScript tricks related to strings to make you more proficient in string manipulation. Let's dive in!
When working with JavaScript strings, there are many interesting techniques that can enhance our coding efficiency. In this article, I will introduce some JavaScript tricks related to strings to make you more proficient in string manipulation. Let's dive in!
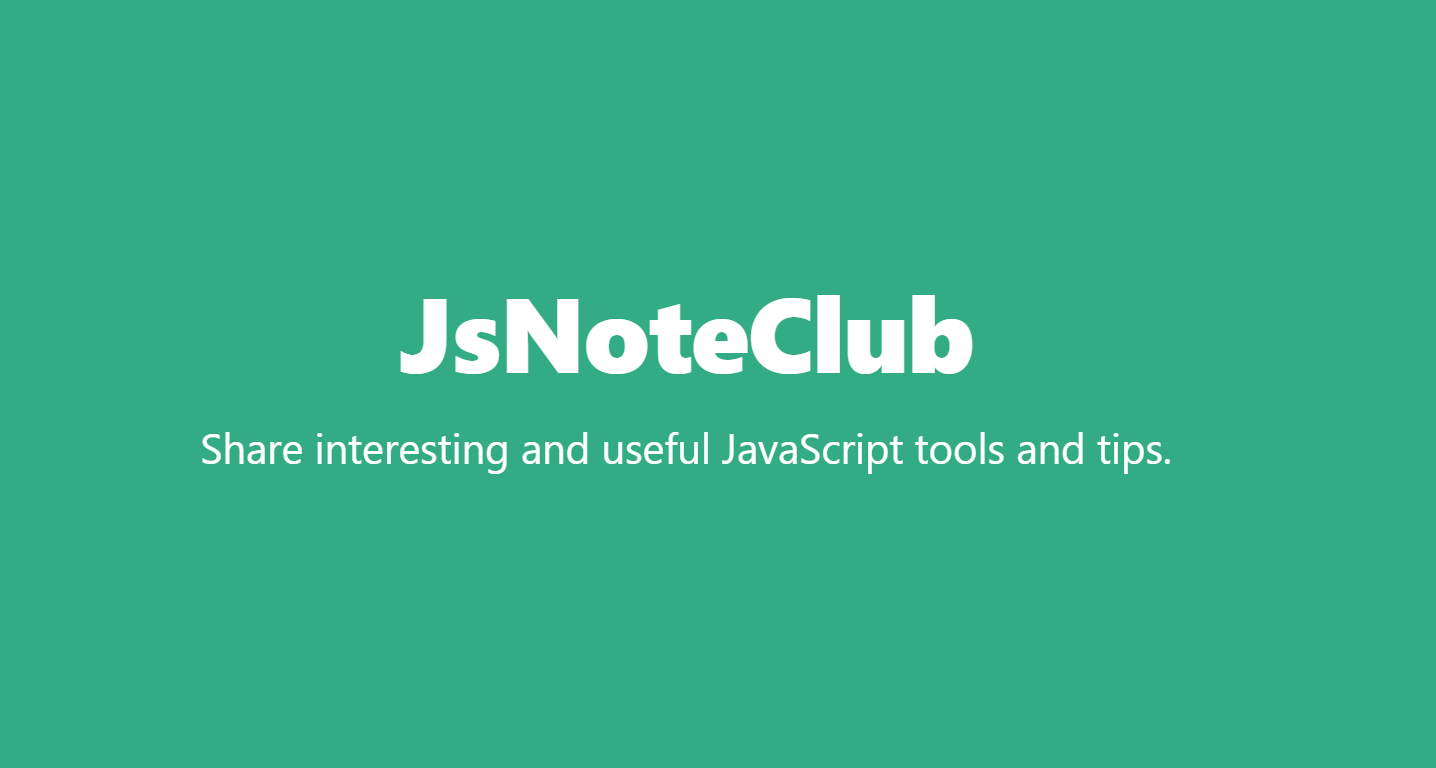
String Padding
Sometimes, we may need to ensure that a string reaches a specific length. This is where the padStart
and padEnd
methods come in handy. These methods are used to pad a specified character at the beginning and end of a string until it reaches the specified length.
// Use the padStart method to pad "0" characters at the beginning of the string until the length is 8
const binary = '101'.padStart(8, '0');
console.log(binary); // "00000101"
// Use the padEnd method to pad "*" characters at the end of the string until the length is 10
const str = "Hello".padEnd(11, " *");
console.log(str); // "Hello * * *"
String Reversal
Reversing characters in a string is a common requirement, and it can be achieved using the spread operator (...
), the reverse
method, and the join
method.
// Reverse the characters in the string, using the spread operator, reverse method, and join method
const str = "developer";
const reversedStr = [...str].reverse().join("");
console.log(reversedStr); // "repoleved"
Capitalize the First Letter
To capitalize the first letter of a string, various methods can be used, such as toUpperCase
and slice
methods or using an array of characters.
// To capitalize the first letter, use toUpperCase and slice methods
let city = 'paris';
city = city[0].toUpperCase() + city.slice(1);
console.log(city); // "Paris"
String to Array Conversion
If you need to split a string into a character array, you can use the spread operator (...
).
// Split the string into a character array using the spread operator
const str = 'JavaScript';
const characters = [...str];
console.log(characters); // ["J", "a", "v", "a", "S", "c", "r", "i", "p", "t"]
Splitting Strings with Multiple Delimiters
Besides regular string splitting, you can use regular expressions to split a string based on multiple delimiters.
// Split string on multiple delimiters using regular expressions and split method
const str = "java,css;javascript";
const data = str.split(/[,;]/);
console.log(data); // ["java", "css", "javascript"]
Checking if a String Contains a Specific Sequence
You can use the includes
method to check if a string contains a specific sequence without using regular expressions.
// Use the includes method to check if a string contains a specific sequence
const str = "javascript is fun";
console.log(str.includes("javascript")); // true
Checking if a String Starts or Ends with a Specific Sequence
If you need to check if a string starts or ends with a specific sequence, you can use the startsWith
and endsWith
methods.
// Use startsWith and endsWith methods to check if a string starts or ends with a specific sequence
const str = "Hello, world!";
console.log(str.startsWith("Hello")); // true
console.log(str.endsWith("world")); // false
String Replacement
To replace all occurrences of a specific substring in a string, you can use regular expression methods with global flags or the new replaceAll
method (note: not supported in all browsers and Node.js versions).
// Use the replace method combined with a regular expression with global flags to replace all occurrences of a string.
const str = "I love JavaScript, JavaScript is amazing!";
console.log(str.replace(/JavaScript/g, "Node.js")); // "I love Node.js, Node.js is amazing!"
Conclusion
JavaScript string manipulation goes beyond simple concatenation and trimming. The 8 techniques introduced in this article are just a part of string operations, and there are many more waiting for you to explore.
These tricks will make you more flexible and efficient when working with strings. I hope these techniques are helpful in your programming journey.
Learn more:
Article Link:https://jsnoteclub.com/blog/11-useful-javascript-tips-to-boost-your-efficiency/
JsNoteClub:Share interesting and useful JavaScript tools and tips.