How to Call JavaScript Functions in HTML Without Using onclick
Discover how to invoke JavaScript functions in HTML without onclick. Employ the flexible addEventListener method for diverse event handling, empowering dynamic front-end interactions and enhancing user experiences.
Calling JavaScript functions in HTML is a common task in front-end development. Typically, the onclick
attribute is used to trigger functions, but sometimes, we want to execute JavaScript functions without relying on user clicks. This article explores how to call JavaScript functions in HTML without using the onclick
attribute, discussing its application scenarios and detailed implementation methods.
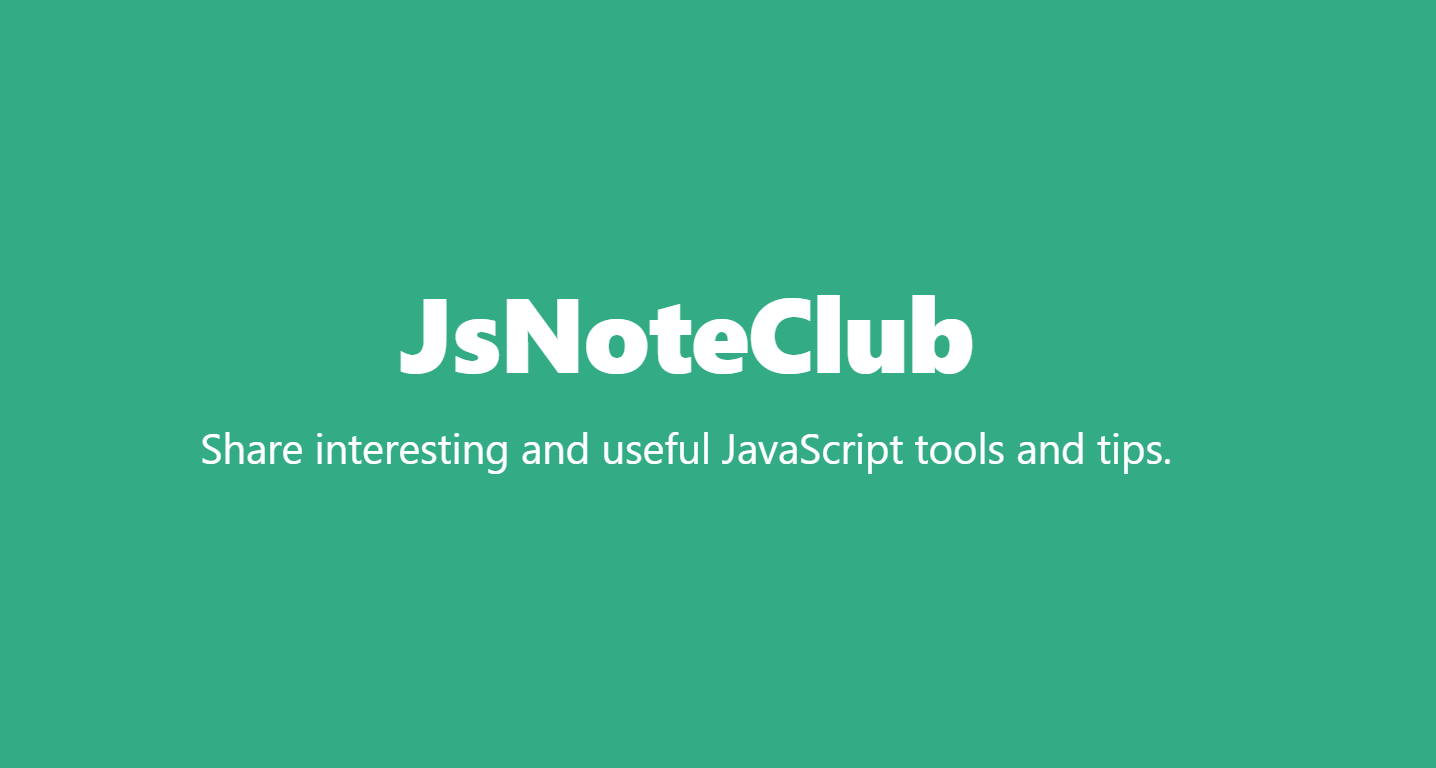
Application Scenarios
- Tasks on Page Load: Execute initialization tasks after the page has loaded, without waiting for a user click.
- Form Validation: Validate forms before submission, not solely relying on the click event of the submit button.
- Scheduled Tasks: Implement functionality triggered at specific intervals, such as carousel image switching or timed reminders.
Overview of the Feature
How to call JavaScript functions in HTML without using onclick
? The main purpose of this feature is to use event listeners to respond to different events, not just user click events. This approach provides more flexibility in controlling when functions are executed.
Syntax and Usage
1. Using addEventListener
Method
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Call JavaScript Functions</title>
</head>
<body>
<button id="myButton">Click Me</button>
<script>
function myFunction() {
console.log("Function has been called!");
}
// Add an event listener
document.getElementById("myButton").addEventListener("click", myFunction);
</script>
</body>
</html>
2. Using Anonymous Function
<script>
document.getElementById("myButton").addEventListener("mouseover", function() {
console.log("Mouse is over the button!");
});
</script>
3. Using Arrow Function
<script>
const myFunction = () => {
console.log("Arrow function has been called!");
};
document.getElementById("myButton").addEventListener("dblclick", myFunction);
</script>
Practical Examples
Example: Execute Tasks on Page Load
In this example, we'll create a scenario where a function is executed when the page loads. This can be useful for performing initialization tasks without waiting for a user action.
HTML File: index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Execute Tasks on Page Load</title>
</head>
<body>
<!-- Placeholder content -->
<h1>Welcome to My Website!</h1>
<script src="script.js"></script>
</body>
</html>
JavaScript File: script.js
// Define the initialization function
function init() {
console.log("Initialization tasks executed on page load!");
// Additional tasks can be added here
}
// Add an event listener to execute the init function when the page loads
window.addEventListener("load", init);
In this example, the init
function contains the tasks that should be executed when the page loads. The window.addEventListener("load", init)
line ensures that the init
function is called when the entire page, including its dependencies, has finished loading.When you open the index.html
file in a web browser, you should see the message "Initialization tasks executed on page load!" in the browser's console. This demonstrates how to use the load
event to trigger JavaScript functions when the page is fully loaded.Feel free to add more tasks to the init
function or experiment with other events to see how they can be used to trigger different actions on page load.
Interactive Exercise
Exercise: Create a Button with Mouseover Event
HTML File: index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Mouseover Event Example</title>
</head>
<body>
<!-- Your button here -->
<button id="hoverButton">Hover over me</button>
<script src="script.js"></script>
</body>
</html>
JavaScript File: script.js
// Your JavaScript code here
// Add an event listener to the button for the mouseover event
document.getElementById("hoverButton").addEventListener("mouseover", function() {
console.log("Mouse is hovering over the button!");
});
Reference Answer
When you hover over the button, you should see the message "Mouse is hovering over the button!" in the browser's console.This exercise demonstrates how to use the mouseover
event to trigger a function when the mouse is moved over a specific element.
Tips, Tricks, or Considerations
- The event type (e.g., "click," "mouseover") determines when the function is executed; choosing the appropriate event type is crucial.
- When using the
addEventListener
method, ensure that the passed function is defined, or it will result in an error. - You can add multiple event listeners of the same type to one element; they will execute in the order they were added.
Conclusion
Through the addEventListener
method, we can call JavaScript functions in HTML without relying on the onclick
attribute. This method allows for more flexible control over when functions are executed and is applicable to various scenarios, including executing tasks on page load, form validation, and scheduled tasks.Hopefully, the knowledge provided in this article will help you better handle the interaction between HTML and JavaScript. Keep learning, keep exploring, and you'll be able to create more powerful and interactive front-end pages.
References
Learn more:
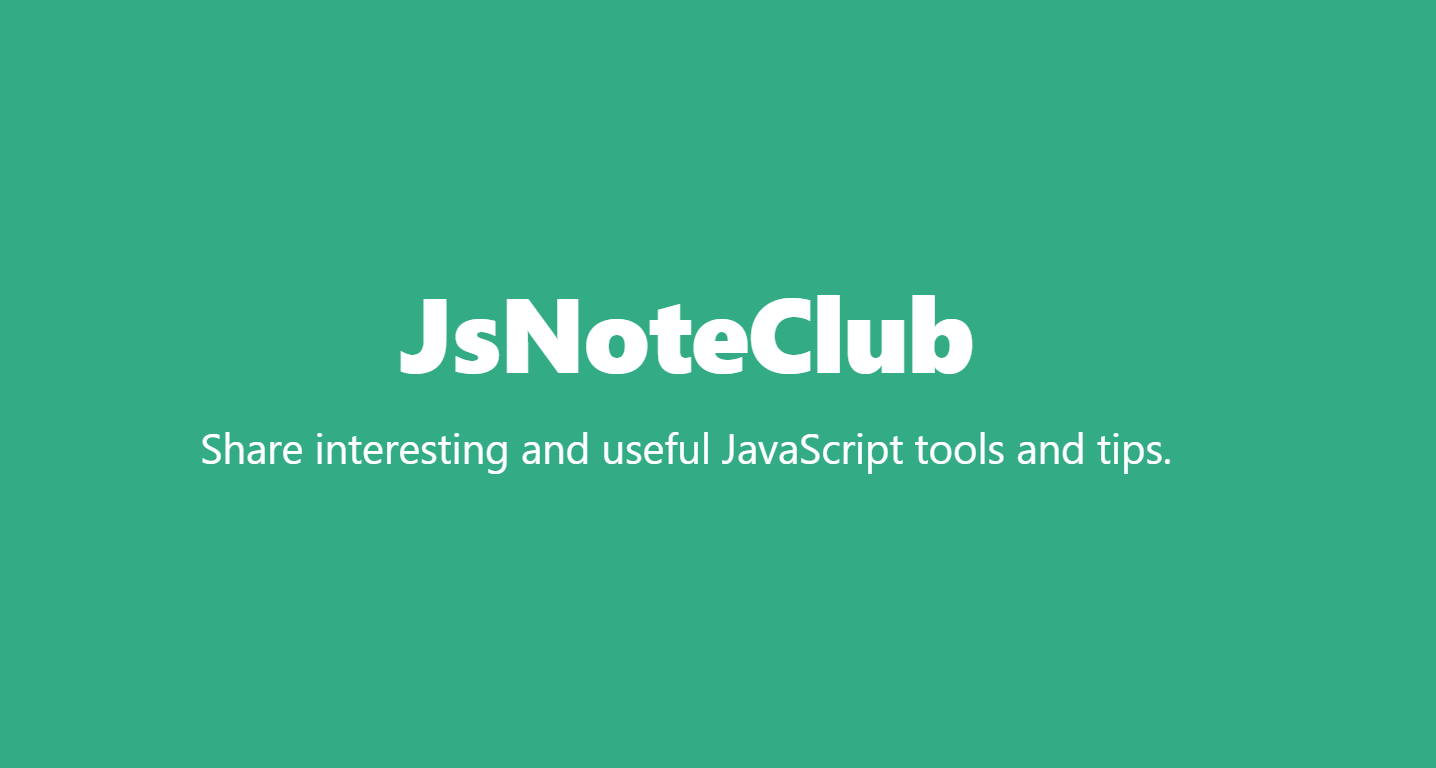
JsNoteClub:Share interesting and useful JavaScript tools and tips.