How to Square Numbers in JavaScript
In JavaScript programming, manipulating numbers is a commonplace task. Squaring numbers is a fundamental mathematical operation, and JavaScript provides several ways to achieve this task. In this article, we will focus on discussing common methods in JavaScript for squaring numbers.
In JavaScript programming, manipulating numbers is a fundamental task, and squaring numbers is a common operation within this context. This article delves into multiple approaches for squaring numbers in JavaScript, introducing different methods such as using the multiplication operator, the exponentiation operator, and the Math.pow() function. By understanding these methods, developers can choose the most suitable approach, enhancing code readability and flexibility. Let's explore these techniques together and deepen our understanding of numerical operations in JavaScript.
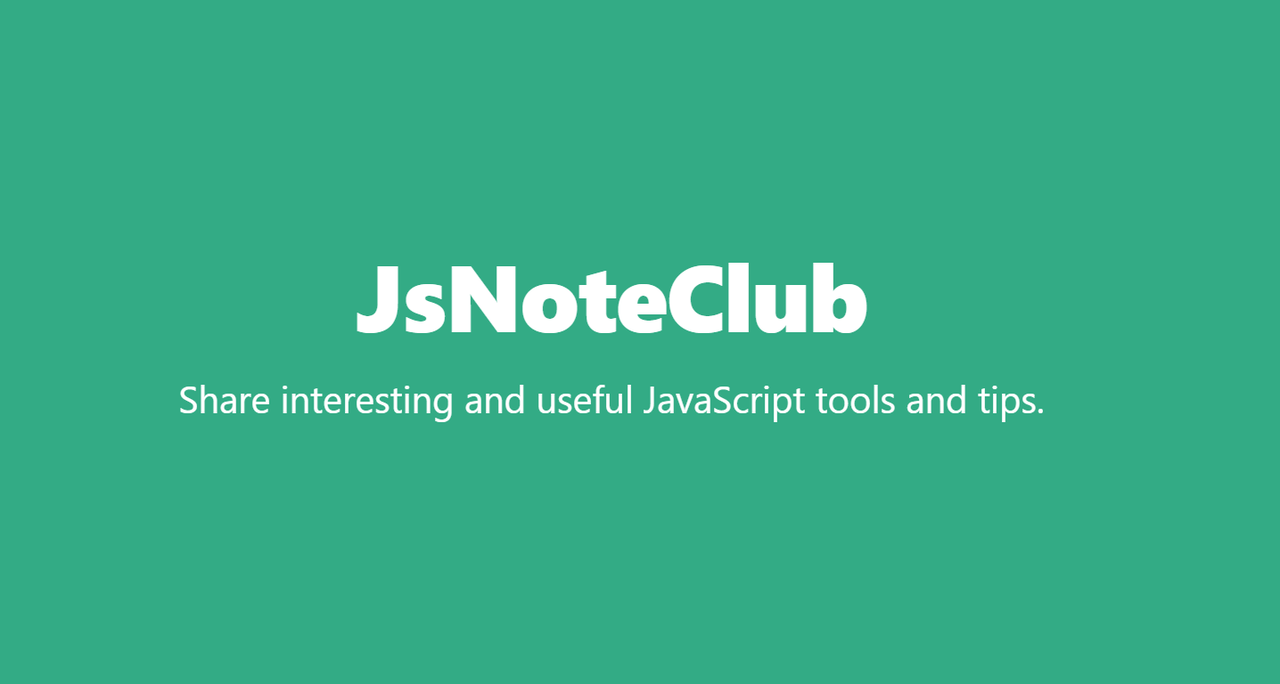
Common Methods
In JavaScript, there are several methods for squaring numbers. Here are some of them:
Multiplication Operator (*
)
The multiplication operator is the most straightforward method, achieving the squaring operation by multiplying a number by itself. For example:
var num = 5;
var square = num * num;
Pros:
- Simple and clear, with minimal code.
- May offer better performance in straightforward scenarios.
Cons:
- For large numbers, there is a potential risk of integer overflow.
Exponentiation Operator (**
)
The exponentiation operator, introduced in the ECMAScript 2016 standard, provides a concise way to perform exponentiation, specifically squaring a number. For example:
var num = 5;
var square = num ** 2;
Pros:
- Concise syntax, enhancing readability.
- Less prone to integer overflow issues.
Cons:
- Not supported in some older versions of JavaScript environments.
Math.pow()
Method
The Math.pow() method is a function provided by the Math object for executing exponentiation. It takes two parameters: the base and the exponent. For example:
var num = 5;
var square = Math.pow(num, 2);
Pros:
- Offers flexibility for arbitrary exponentiation, not limited to squaring.
- Particularly useful for complex mathematical calculations.
Cons:
- Relatively cumbersome syntax, requiring a method call.
- In simpler scenarios, performance may be slightly inferior to the multiplication operator.
Comparison
- Conciseness:
- The multiplication operator and exponentiation operator are more concise in terms of syntax, especially the exponentiation operator.
- Math.pow() method is relatively more verbose, involving method calls and parameter passing.
- Performance:
- In simple scenarios, the multiplication operator may have superior performance.
- In complex mathematical calculations, Math.pow() method provides greater flexibility.
- Functionality:
- Math.pow() method is the most versatile, capable of arbitrary exponentiation beyond squaring.
The choice of method depends on specific requirements and context. In simpler cases, the multiplication operator or exponentiation operator may be more suitable, while in situations requiring greater flexibility and functionality, the Math.pow() method stands out as the preferred choice.
Conclusion
In summary, JavaScript offers developers multiple methods for squaring numbers, each with its unique advantages. The choice of method depends on personal preferences, code context, and performance requirements. By mastering these methods, developers can handle numerical operations more flexibly and write code that is clearer and more efficient. It is our hope that this article assists you in gaining a better understanding of squaring numbers in JavaScript and aids in selecting the most suitable method for practical development.
Reference Links:
Learn more:
- How to Create a Grid of Divs Using JavaScript
- 30 JavaScript One-Liners to Turn You into a JavaScript Wizard
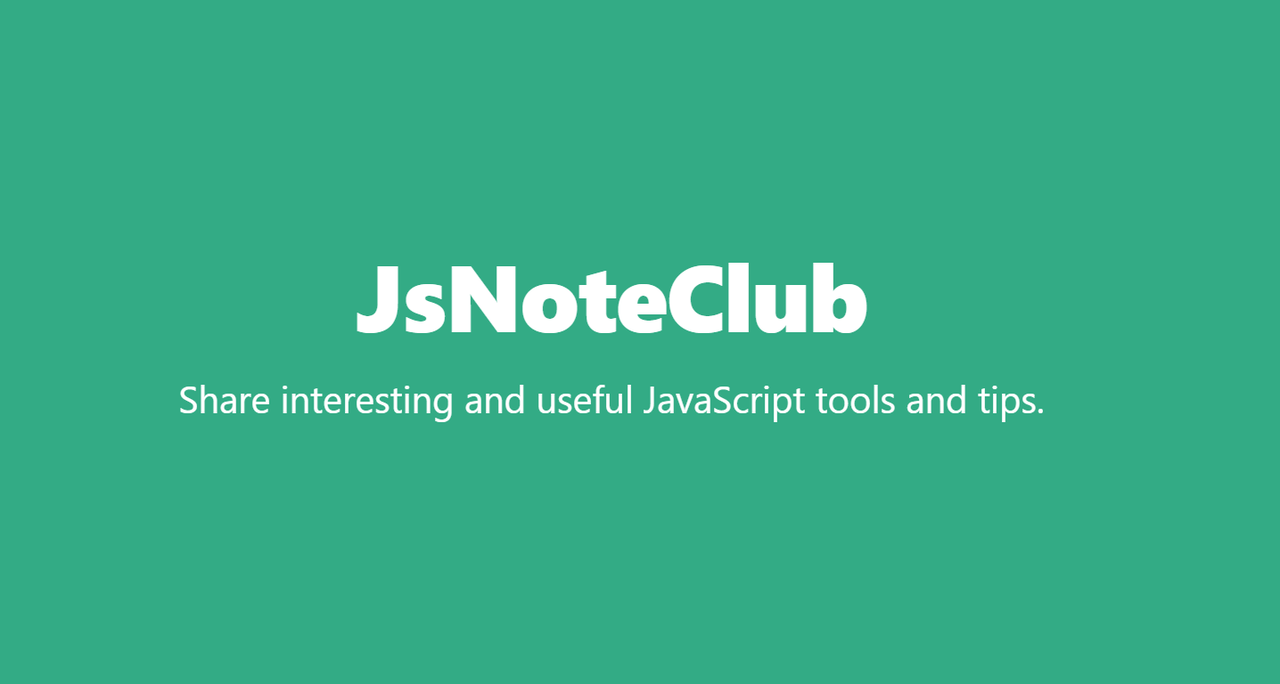